Publish Date - December 15th, 2022
|Last Modified - March 7th, 2023
A great SQL problem, that needs a high level of attention to detail. This problem is not as technically complicated as some of the previous problems like Draw the Triangle 1 or Draw the Triangle 2. The use of CONCAT is advised for this challenge, amongst other functions because of the required output suggested by the author.
The problem
Generate the following two result sets:
- Query an alphabetically ordered list of all names in OCCUPATIONS, immediately followed by the first letter of each profession as a parenthetical (i.e.: enclosed in parentheses). For example:
AnActorName(A)
,ADoctorName(D)
,AProfessorName(P)
, andASingerName(S)
. - Query the number of ocurrences of each occupation in OCCUPATIONS. Sort the occurrences in ascending order, and output them in the following format:
There are a total of [occupation_count] [occupation]s. where [occupation_count] is the number of occurrences of an occupation in OCCUPATIONS and [occupation] is the lowercase occupation name. If more than one Occupation has the same [occupation_count], they should be ordered alphabetically.
Note: There will be at least two entries in the table for each type of occupation.
Input Format
The OCCUPATIONS table is described as follows:
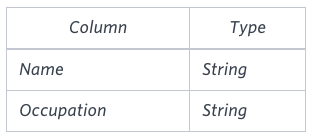
Occupation will only contain one of the following values: Doctor, Professor, Singer or Actor.
Sample Input
An OCCUPATIONS table that contains the following records:
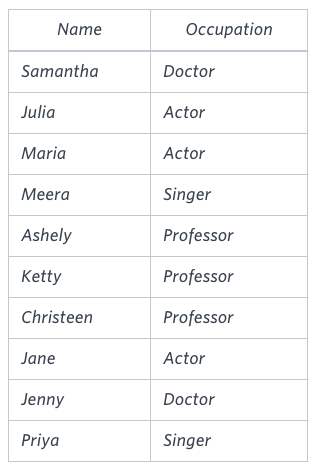
Sample output
Ashely(P)
Christeen(P)
Jane(A)
Jenny(D)
Julia(A)
Ketty(P)
Maria(A)
Meera(S)
Priya(S)
Samantha(D)
There are a total of 2 doctors.
There are a total of 2 singers.
There are a total of 3 actors.
There are a total of 3 professors.
Explanation
The results of the first query are formatted to the problem description’s specifications.
The results of the second query are ascendingly ordered first by number of names corresponding to each profession (2 <= 2 <= 3 <= 3), and then alphabetically by profession ( doctor <= singer, actor <= professor).
The solution
Here’s the output that you’re looking for!
Aamina(D)
Ashley(P)
Belvet(P)
Britney(P)
Christeen(S)
Eve(A)
Jane(S)
Jennifer(A)
Jenny(S)
Julia(D)
Ketty(A)
Kristeen(S)
Maria(P)
Meera(P)
Naomi(P)
Priya(D)
Priyanka(P)
Samantha(A)
There are a total of 3 doctors.
There are a total of 4 actors.
There are a total of 4 singers.
There are a total of 7 professors.
Note the following things about this output:
- The order in which the names appear
- brackets on the actual professions
- Spacing in the final 4 lines of output
- pluralization and punctuation of the queries in the last 4 outputs
Here’s my query (Updated for March 2023)
/*
1. ORDER BY Names in OCCUPATIONS followed by the profession's first letter
2. COUNT of each Occuption count ORDER BY ASC, with the output There are a total of [occupation_count] [occupation]s. If there's a tie, ORDER BY occuption
*/
SELECT CONCAT(Name, “(“, LEFT(occupation,1), “)”) AS a FROM occupations
UNION
SELECT CONCAT(“There are a total of “, COUNT(occupation),” “, lower(occupation),”s”,”.”)
FROM occupations
GROUP BY occupation
ORDER BY a
As always I start by writing sudo code so that you can convert that more easily into actual code. Now, CONCAT is the most important portion of this answer because it will help you string all of your content together. Since this problem asks for two queries, I broke it up into two queries (woe is me because I thought I’d have to UNION the two queries together originally).
Section One:
SELECT CONCAT(Name, "(", LEFT(occupation,1), ")") AS a FROM occupations
/* This is the first half of the query, where you will pull the name - the first letter of every record in Occupation with respect to that name in bracket. REMEMBER, to order by Name so that you end up getting the alphabetical output of names (as requested in the problem) you will need the alias at the end to be able to use the union.*/
If you just query this above, you’ll get this!
Aamina(D)
Ashley(P)
Belvet(P)
Britney(P)
Christeen(S)
Eve(A)
Jane(S)
Jennifer(A)
Jenny(S)
Julia(D)
Ketty(A)
Kristeen(S)
Maria(P)
Meera(P)
Naomi(P)
Priya(D)
Priyanka(P)
Samantha(A)
Pretty easy right?
Section Two:
SELECT CONCAT(“There are a total of “, COUNT(occupation),” “, lower(occupation),”s”,”.”)
FROM occupations
GROUP BY occupation
ORDER BY a
/* Note the CONCAT with the lower case on the occupation, the spacing, the "s" and the ".". This is so that you can get that nice string that's requested in the end. (EX: There are a total of 3 doctors.). From there, you'll need to GROUP BY because you're aggregating and then because you've made a virtual column with the first query (a) you don't need to make the change.*/
Conclusion
Great question overall that had be overthinking once again. After all of my attempts at trying to SET a variable for and do a self / sub-query, I realized I could just string concatenate.. Occam’s Razor – sometimes the best approach is the simplest!
Check out some of my guides for Udemy, where I learned to code!
I have a question that i want to ask you, Why doesn’t the second select statement need an “order by count(occupation)” clause and it can still sort the results in ascending order? I’m looking forward to your response. Thanks
Hey Manh,
Thanks for reaching out. By second statement, I’m assuming you mean this query:
SELECT CONCAT(“There are a total of “, COUNT(occupation),” “, lower(occupation),”s”,”.”)
FROM occupations
GROUP BY occupation
ORDER BY a
The reason it works is the following:
When I create the alias “a” in the first statement, it’s giving my a virtual column which I can order by. Because I’ve done a UNION and a GROUP BY, there’s a mapping from the first query to the second query that makes the second query dependent on the first query.
Based on the very first query and it’s output, MYSQL will basically group the first value it sees and continue to go down the list (similar to a FOR LOOP doing i += 1).
Ashley(P) (PROFESSOR +1)
Samantha(A) (ACTOR +1)
Julia(D) (DOCTOR +1)
Britney(P) (PROFESSOR +2)
Maria(P) (PROFESSOR +3)
Meera(P)(PROFESSOR +4)
Priya(D) (DOCTOR +2)
Priyanka(P) (PROFESSOR +5)
Jennifer(A) (ACTOR +2)
Ketty(A) (ACTOR +3)
Belvet(P) (PROFESSOR +6)
Naomi(P) (PROFESSOR +7)
Jane(S) (SINGER +1)
Jenny(S) (SINGER +2)
Kristeen(S) (SINGER +3)
Christeen(S) (SINGER +4)
Eve(A) (ACTOR +4)
Aamina(D) (DOCTOR +3)
So the ORDER of the group is (PROFESSOR, DOCTOR, ACTOR, SINGER). Which is what you will see if you query w/o the ORDER BY clause in second statement.
By default, MySQL should be doing ascending order, but there is no order because the GROUP BY is pivoting on a custom alias.
Therefore, when I slap that ORDER BY a – it reorganizes the WHOLE QUERY into ascending order, not just the last values.
Hope this helps!
Hey Mohd!
I see the problem, it’s odd because I actually submitted the answer a few months ago and it passed the submission test case.
https://yourdigitalaid.com/wp-content/uploads/2023/03/submissions-passed.png
Anyways, just use an alias for the first query, so that you can use order by the newly constructed column. Add union and then order by after.
Hope this helps!
SELECT CONCAT(Name, “(“, LEFT(occupation,1), “)”) AS a FROM occupations
UNION
SELECT CONCAT(“There are a total of “, COUNT(occupation),” “, lower(occupation),”s”,”.”)
FROM occupations
GROUP BY occupation
ORDER BY a
I tried both queries you uploaded but How to merge both queries can you please let me know?
I tried Union keyword but Can’t use Union keyword with Order by clause